Example: Updating an API's Documentation Using GitLab (GQL Platform API)
Automatically update an API's documentation in Rapid when a README.md file is updated in a GitLab repository.
This example shows how to integrate and automate a Rapid Enterprise Hub and a GitLab repository. When a change is made to the README.md file in a GitLab repository, the associated API's Documentation will automatically be updated in Rapid.
Overview
The following basic steps can be used to set up a CI/CD pipeline in GitLab to automatically update an API's documentation in Rapid when a change is made to the API's README.md file in a GitLab repository.
- Create a GitLab CI/CD pipeline .yaml file
- Create a script to update the API's documentation in Rapid
- Define environment variables in GitLab
- Test the integration
Create a GitLab CI/CD pipeline .yaml file
The following code configures a GitLab CI/CD pipeline job that would be automatically triggered whenever a change is made within the GitLab repository. For simplicity, we have split the script used to update the API documentation in Rapid (/scripts/update-readme.js) rather than embedding the code within the pipeline yaml.
Note: We have included βmockβ deploy stages [which are NOT required] to illustrate how publishing to Rapid will fit into a broader deployment pipeline. The main function is update-read-me-rapid
for this example
image: node:latest
stages: # List of stages for jobs, and their order of execution
- deploy
- publish
# PLACEHOLDER - NOT FUNCTIONAL UNIT TESTING
deploy-job: # This job runs in the deploy stage.
stage: deploy # It only runs when *both* jobs in the test stage complete successfully.
environment: production
script:
- echo "Deploying application..."
- echo "Application successfully deployed."
## THIS IS THE PRIMARY CI CODE FOR RAPID
update-read-me-rapid: # This job runs in the deploy stage.
stage: publish # It can run at the same time as publish-to-rapid (in parallel).
environment: production
Script:
- npm install axios
- npm install fs
- node scripts/update-readme.js
Create a script to update the API's documentation in Rapid
Node.js example
The script below uses Node.js. Any modern programming or scripting language can be used instead.
Now that we have the pipeline job defined, we need to create the script to execute the code that calls Rapidβs Enterprise Hub.
This code is calling the Rapid GQL Platform API. The primary steps in this function are:
- Read the README.md file using
fs
. - Escape the protected characters like quotes and new lines.
- Call the createDocumentation mutation of the GQL Platform API. This mutation can be used to create, update, or delete the documentation.
const axios = require('axios');
constβ―fsβ―=β―require('fs');
try {
var data = fs.readFileSync('README.md', 'utf8');
// REGEX to escape double quotes
data = data.replace(/"/g, '\\"')
// escape newline characters
data = data.split('\n').join('\\n');
const options = {
method: 'POST',
url: 'https://graphql-platform.p.rapidapi.com/',
headers: {
'x-rapidapi-key': process.env.RAPID_API_KEY,
'x-rapidapi-host': process.env.RAPID_GQL_HOST,
'x-rapidapi-identity-key': process.env.RAPID_IDENTITY_KEY,
'Content-Type': 'application/json'
},
data: {
query: `mutation CreateDocumentation { createDocumentation(input: {apiId: "` + process.env.RAPID_API_ID + `", readme: "`+ data +`"})}`
}
};
console.log(options);
axios(options)
.then(function (response) {
console.log(response.data);
});
} catch(e) {
console.log('Error:', e.stack);
}
Define environment variables in GitLab
For security reasons, we have put the GraphQL Platform API host and API key into environment variables. We have also put the API ID into an environment variable to easily port this script from one repository to another.
To update these environment variables in GitLab, go to Project > Settings > CI/CD > Variables. Here you can save the variables:
- RAPID_API_ID: The ID of the API you want to update in Rapidβs Enterprise Hub.
- RAPID_API_KEY: The API key (either personal account or team) that is approved for access to the GraphQL Platform API.
- RAPID_IDENTITY_KEY: The API key of your personal account within Rapidβs Enterprise Hub
- RAPID_GQL_HOST: The host of the GraphQL Platform API for your tenant of the Enterprise Hub
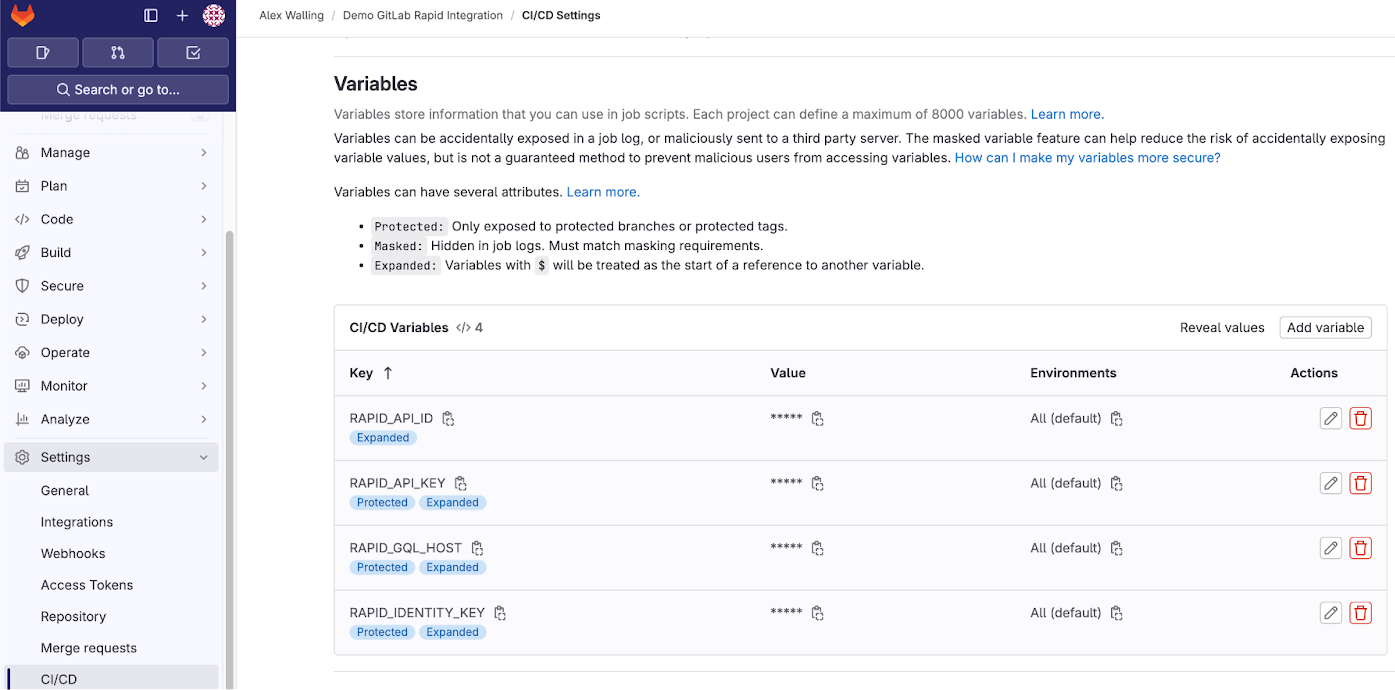
Setting environment variables in GitLab.
Test the integration
Your GitLab CI/CD pipeline job is ready to test. Simply publish your changes to the repository and see the code in action:
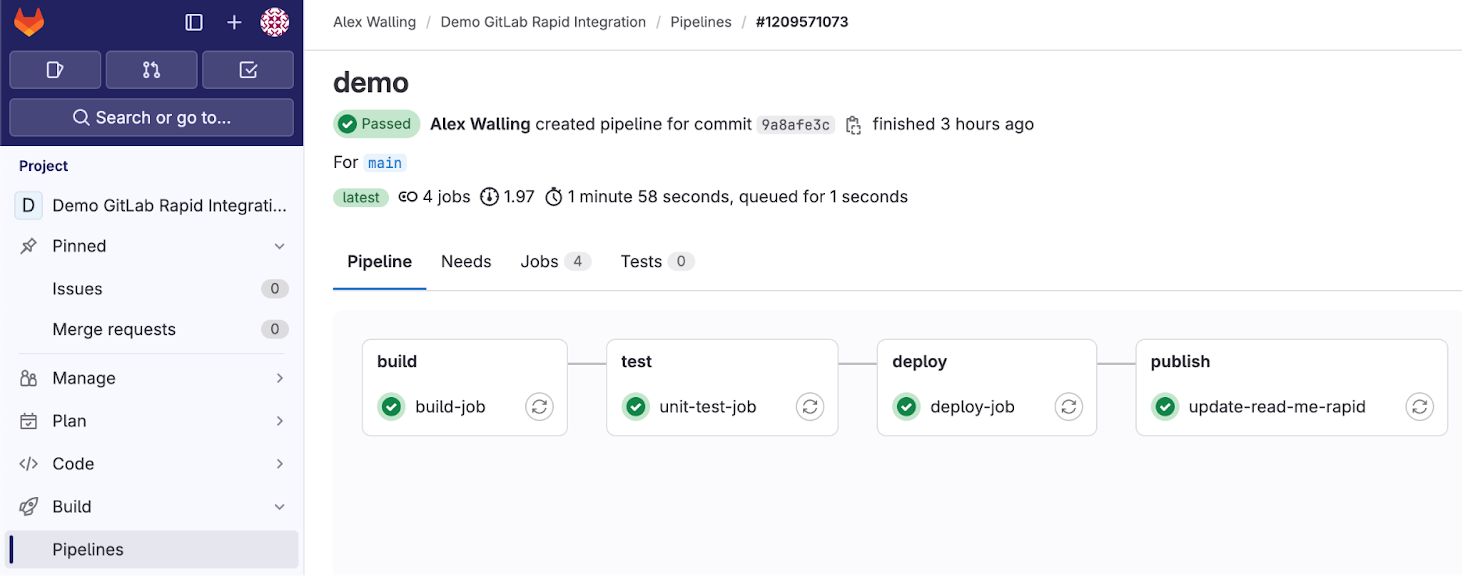
Testing the pipeline in GitLab.
Updated about 1 month ago