Reading API Information (GQL PAPI)
Obtain an API's details
- To query for an API's details, you must add an id argument to the query. This is so the server knows which API's details to return. Paste the following query in the GQL Query textbox in the RapidAPI playground (API Hub), replacing the id with any valid API's id. You can obtain an API id using Studio (My APIs > Hub Listing (for any API) > Endpoints > OpenAPI > API Details). You can also obtain the id programmatically .
If the API is private, you must make this query using a context that has access to the API (either as an owner or by being invited to use the API). If the private API is owned by a team, you must include the
x-rapidapi-identity-key
header. See GraphQL Platform API Authorization for more information.
query {
api(id: "api_1713daa7-32ff-473b-b98d-xxxxxxxx") {
id
name
slugifiedName
visibility
description
longDescription
documentation {
readme {
text
}
spotlights {
spotlightURL
status
title
description
}
}
ownerId
owner {
name
slugifiedName
}
currentVersion {
name
id
}
versions {
id
name
targetGroup {
loadBalancingStrategy
id
targetUrls {
url
}
}
}
}
}
-
Click Test Endpoint. You should see a response with details of the API. Details about the query and the response are described next.
-
Details about the query:
-
In the left frame, expand the Queries operation.
-
Scroll down and click api.
-
You should see that the
api
query expects an argument of id. This was specified in the query above. -
Notice the FIELDS that you can query for. In the query above, we queried for the name, slugifiedName, visibility, and currentVersion fields.
-
Click the currentVersion field. This brings you to the description of an object of type ApiVersion. Notice that this type includes the name and id fields that are specified under currentVersion in the query above. This is one of the advantages of GraphQL APIs - you can query for data from multiple types in a single query. This is typically done with more than one query in REST APIs.
-
-
Details about the code snippet:
-
In the right frame, click Code Snippets.
-
Notice that the request is a POST request to a single endpoint (the root endpoint). All of the GraphQL requests are made to this single endpoint.
-
Notice the data object. It contains a query object containing the query specified in the middle frame's GQL Query textbox.
-
-
Details about the response:
- Expand the details of the response by clicking Results and verify that you have received values in a format that matches the query. This includes details of the currentVersion object.
-
(Optional) The query above βhard-codesβ the api id in the GraphQL document. This is usually not recommended, because the query is not reusable. Change the query to define and use an $apiId variable:
query readAPI($apiId: ID!) {
api(id: $apiId) {
id
name
slugifiedName
visibility
ownerId
owner {
name
slugifiedName
}
currentVersion {
name
id
}
versions {
id
name
targetGroup {
loadBalancingStrategy
id
targetUrls {
url
}
}
}
}
}
- To assign a value to the $apiId variable, click on the variables button below the GQL Query textbox and enter the following (you will need to change the value of apiId):
{
"apiId": "api_15d502af-e297-4f9d-b275-xxxxxxxxxx"
}
- Click Test Endpoint and verify that the query still functions as before. Notice that the variable assignment is included in the code snippet.
- Modify the query to add another field. For example, you could add
category
to the query. Test your results.
Here is some sample code using the Axios library and Node.js. You must enter valid values for url, x-rapidapi-host , x-rapidapi-key and the apiId in the variables. These can all be obtain from the Code Snippets on the right of the API Playground.
const axios = require("axios");
const options = {
method: "POST",
url: "https://xxx-graphql.p.rapidapi.com/",
headers: {
"content-type": "application/json",
"X-RapidAPI-Key": "b1af91e76emsh70b8551086xxxxx",
"X-RapidAPI-Host": "platform-xxx.xxx-training.rapidapi.com",
},
data: {
query: `query readAPI($apiId: ID!) {
api(id: $apiId) {
id
name
slugifiedName
visibility
ownerId
owner {
name
slugifiedName
}
currentVersion {
name
id
}
versions {
id
name
}
}
}`,
variables: {
"apiId": "api_2c5487d7-1xxx"
},
},
};
axios
.request(options)
.then(function (response) {
console.log(JSON.stringify(response.data));
})
.catch(function (error) {
console.error(error);
});
Query APIs
List APIs visible to the current calling context
Where input
This query accepts an optional where argument. This argument is of type ApiWhereInput (see the type definition below) and is used to filter the results. If no where argument is specified, this query returns all public APIs and any private APIs visible in the calling context. The calling context may own the private API, or may have been invited to use the API as a consumer.
Pagination
Because this query can return multiple results, it uses pagination. See GraphQL Platform API Pagination for more information.
query {
apis(
where: {
ownerId: 5713300
},
pagination: {
first: 5
},
) {
edges {
node {
id
name
}
}
pageInfo {
endCursor
hasNextPage
}
totalCount
}
}
Here the query uses Variables instead of hard-coding the where value. Click the Variables tab below to see them. Click the ApiWhereInput Type Definition tab to see its type definition.
query apis($where: ApiWhereInput, $orderBy: ApiOrderByInput, $pagination: PaginationInput) {
apis(where: $where, orderBy: $orderBy, pagination: $pagination) {
edges {
node {
id
name
}
}
pageInfo {
endCursor
hasNextPage
}
totalCount
}
}
{
"where": {
"name": [
"MY API NAME"
],
"ownerId": [
"6028339"
]
},
"pagination": {
"first": 5
}
}
type ApiWhereInput {
id: [ID!]
ownerId: [ID!]
subscriberId: [ID!]
visibility: ApiVisibility
apiSlugifiedName: [String!]
ownerSlugifiedName: [String!]
name: [String!]
isFavorite: Boolean
}
List all public or private APIs
This query lists all of the public APIs in the API Hub. Changing the visibility variable to "PRIVATE" lists all private APIs in the API Hub that are visible to the logged in user.
query queryApis ($visibility: ApiVisibility! ) {
apis(
where: {
visibility: $visibility
},
pagination: {
first: 50
},
) {
edges {
node {
id
name
visibility
}
}
pageInfo {
endCursor
hasNextPage
}
totalCount
}
}
{
"visibility": "PUBLIC"
}
{
"visibility": "PRIVATE"
}
List APIs owned by any user or team (admin)
Environment Admins can query for the list of APIs owned by a user or team. Among other places, this query is used in the Users tab of the Admin Panel. In the Variables, the ownerId
array can contain user and/or team IDs.
Environment Admin from personal account
This query only works if you are an Environment Admin. Currently, you must execute this query from your Personal Account (not a team account). This means if the GraphQL Platform API is private, you must personally be invited to use the API.
query apis($where: ApiWhereInput) {
apis(where: $where){
nodes {
id
name
visibility
category
createdAt
}
}
}
{
"where": {
"ownerId": ["5755579"]
}
}
Find APIs by search term
This query returns all public APIs and any private APIs visible in the calling context that contain the search term.
Where input
This query accepts an optional where argument. The term field is required to successfully execute this query. Other fields of SearchAPIWhereInput (see the type definition below), such as exclude and categoryName are used to further filter the results.
Pagination
Because this query can return multiple results, it uses pagination. See GraphQL Platform API Pagination for more information.
query SearchApis($where: SearchApiWhereInput!, $pagination: PaginationInput!, $orderBy: SearchApiOrderByInput) {
searchApis(where: $where, pagination: $pagination, orderBy: $orderBy) {
edges {
node {
name
}
}
pageInfo {
hasNextPage
hasPreviousPage
startCursor
endCursor
}
total
}
}
{
"where": {
"term": "email"
},
"pagination": {
"first": 3
}
}
type SearchApiWhereInput {
categoryNames: [String]
exclude: [String]
term: String!
tags: [Any]
collectionIds: [String]
privateApisJwt: String
locale: Locale
}
Export an OAS document for an API
This query returns the OpenAPI 3.x specification document for an API. See Adding and Updating OpenAPI Documents for more information on RapidAPI's OAS extensions.
The query must include the apiId value and optionally can include the apiVersionId. If the apiVersionId is not included, the API's current version is returned. See Versioning Your API for more information on versions.
A non-null result would be returned only if the target apiId
or apiVersionId
(if specifically provided) have some match in the platform, and either:
- the target API is defined with
PUBLIC
visibility, or - the target API is defined with
PRIVATE
visibility and either the requestor or any team they are a member of is owning the target API or the requestor or any team they are a member of is invited to the target API. If the API is visible to the requestor for export but not owned by it, certain sensitive fields (e.gservers
array) in the exported document may be returned blank.
query (
$apiId: ID!
$apiVersionId: ID
) {
exportOpenApi(
apiId: $apiId
apiVersionId: $apiVersionId
)
}
{
"apiId": "your_api_id",
"apiVersionId": "your_api_version_id"
}
The query returns the OAS document as JSON in the data.exportOpenAPI object (see the screenshot below).
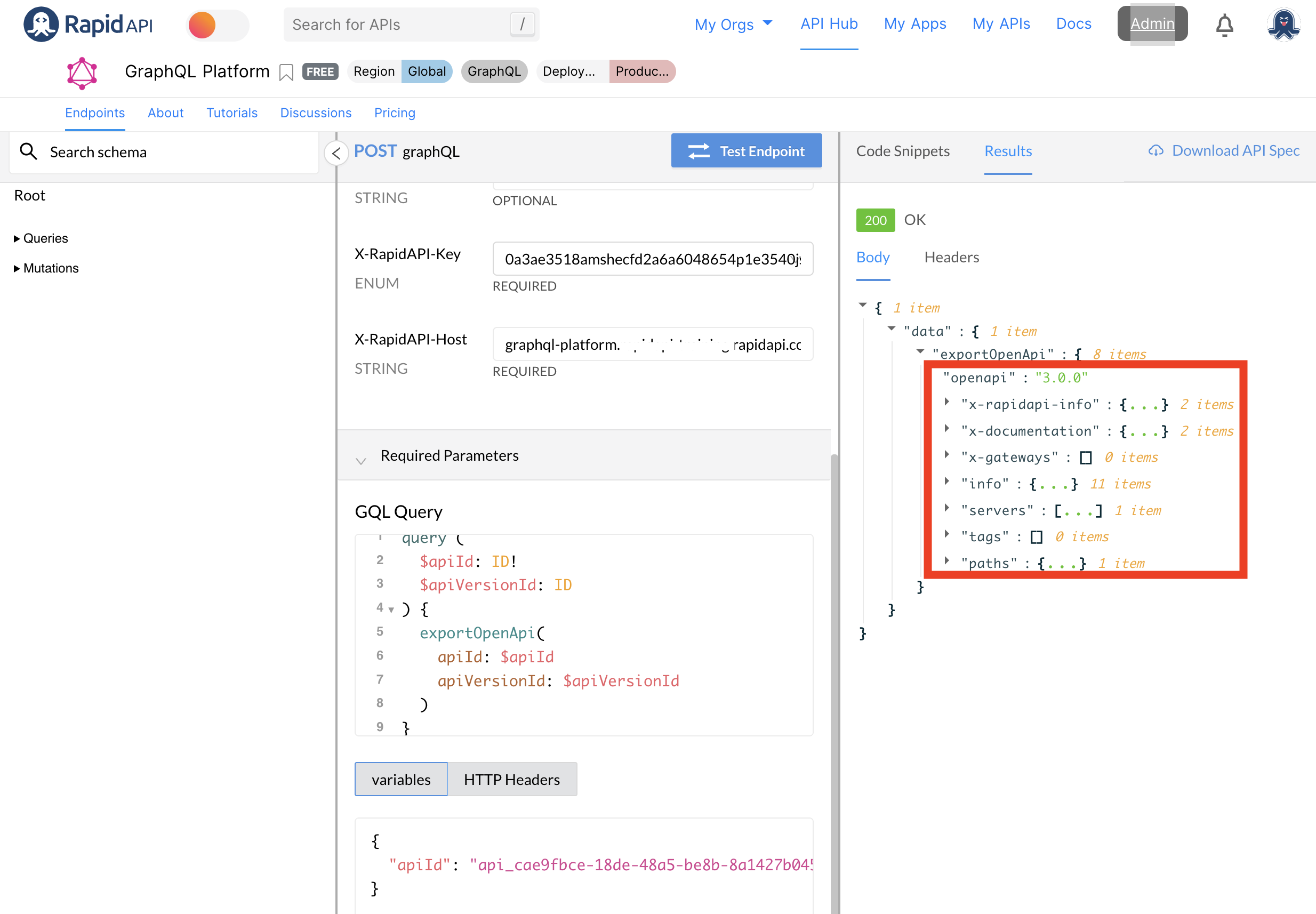
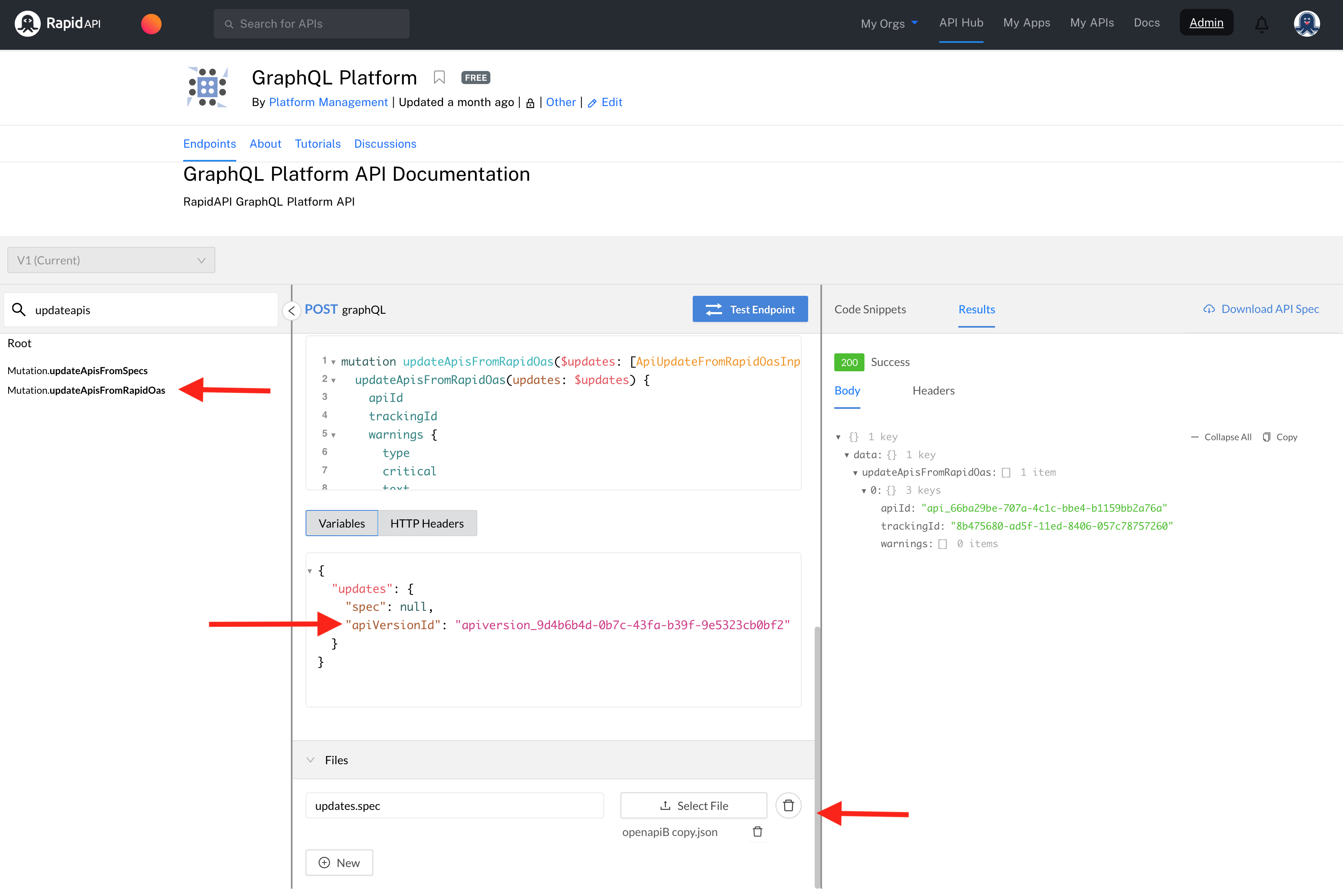
Query an API's versions
Obtains information on all unique versions of an API. The status
field returns the version's lifecycle state ("DRAFT", "ACTIVE", "DEPRECATED"). The endpoints.id
field displays the unique ID for each endpoint of each version of the API.
The API ID of the API you want to query must be included in the variables.
query apiVersions($where: ApiVersionWhereInput) {
apiVersions(where: $where) {
nodes {
id
name
status
current
endpoints {
id
}
}
}
}
{
"where": {
"apiId": "some-api-id"
}
}
Obtain an API's request logs
For details, see API Traffic Logs.
Updated 11 days ago