GraphQL APIs (GQL PAPI)
Create a GraphQL API and read the schema via introspection
If you want to create a GraphQL API and have Rapid read the GraphQL schema using introspection, enter the following variables for the createApi
mutation. The schemaSource
object contains a field with type
introspection
. If you want the introspection to occur only once, set the enableSchemaIntrospectionOnHub
option to false
.
mutation createApi($apiCreateInput: ApiCreateInput!) {
createApi(api: $apiCreateInput) {
id
}
}
{
"apiCreateInput": {
"name": "graphql example",
"category": "Other",
"description": "graphql",
"version": {
"spec": {
"gql": {
"baseUrl": "https://graphqlzero.almansi.me/api",
"schemaSource": {
"type": "introspection",
"options": {
"enableSchemaIntrospectionOnHub": false
}
}
}
}
}
}
}
Create a GraphQL API and include the schema
If you want to create a GraphQL API and include the GraphQL schema in the apiCreateInput
variable, enter the following variables for the createApi
mutation. The schemaSource
object contains a field with type
SDL
and a source
field containing a string representation of the schema. If your GraphQL schema is in a file, your code can read this file and add the schema string to the variables. (The value of source
should be a string on one line - it is formatted differently below for clarity.)
mutation createApi($apiCreateInput: ApiCreateInput!) {
createApi(api: $apiCreateInput) {
id
}
}
{
"apiCreateInput": {
"name": "graphql example",
"category": "Other",
"description": "...",
"version": {
"spec": {
"gql": {
"baseUrl": "https://test.com",
"schemaSource": {
"type": "SDL",
"source": "type Query { getItem: Item } type Mutation { setItem (id: ID name: String): Item } type Item { id: ID name: String }"
}
}
}
}
}
}
Creating an API in a team context
To create an API in a team context, you must include the x-rapidapi-identity-key header parameter. This is used to identify the user creating the API, and to verify that they have the permission to create the API. The value of this header parameter is an app key from your Personal Account. You can obtain this key by selecting your Personal Account when testing any APIβs endpoint and copying the X-RapidAPI-Key header parameter value.
See GraphQL Platform API Authorization for more information.
Update a GraphQL API's introspection URL
See Update an API's base URL. Updating a GraphQL API's Base URL also updates its introspection URL.
Update a GraphQL API's schema
Updating a GraphQL API's other information
This
updateGraphQLSchema
mutation only updates the GraphQL schema for the API. To update other information, such as the API's category, use the updateApi mutation.
Use the updateGraphQLSchema
mutation if you want to update a GraphQL API's GraphQL schema and include the schema in the mutation. The Variables section requires an endpointId
or graphQLSchemaId
(if you include both, the graphQLSchemaId
is prioritized). The endpointId
is the POST endpoint for your GraphQL API and can be obtained using query.apiVersions.
mutation updateGraphQLSchema($input: UpdateGraphQLSchemaInput!) {
updateGraphQLSchema(input: $input)
}
{
"input": {
"endpointId": "apiendpoint_YOUR ENDPOINT ID OBTAINED FROM query.apiVersions"
}
}
You can use the API Hub playground to execute this mutation. In the left frame, highlight the Mutation.updateGraphQLSchema
operation and click ADD QUERY, as shown in the screenshot below. In addition to adding the Variables, scroll down to the Files area and select the local file containing your API's updated GraphQL Schema.
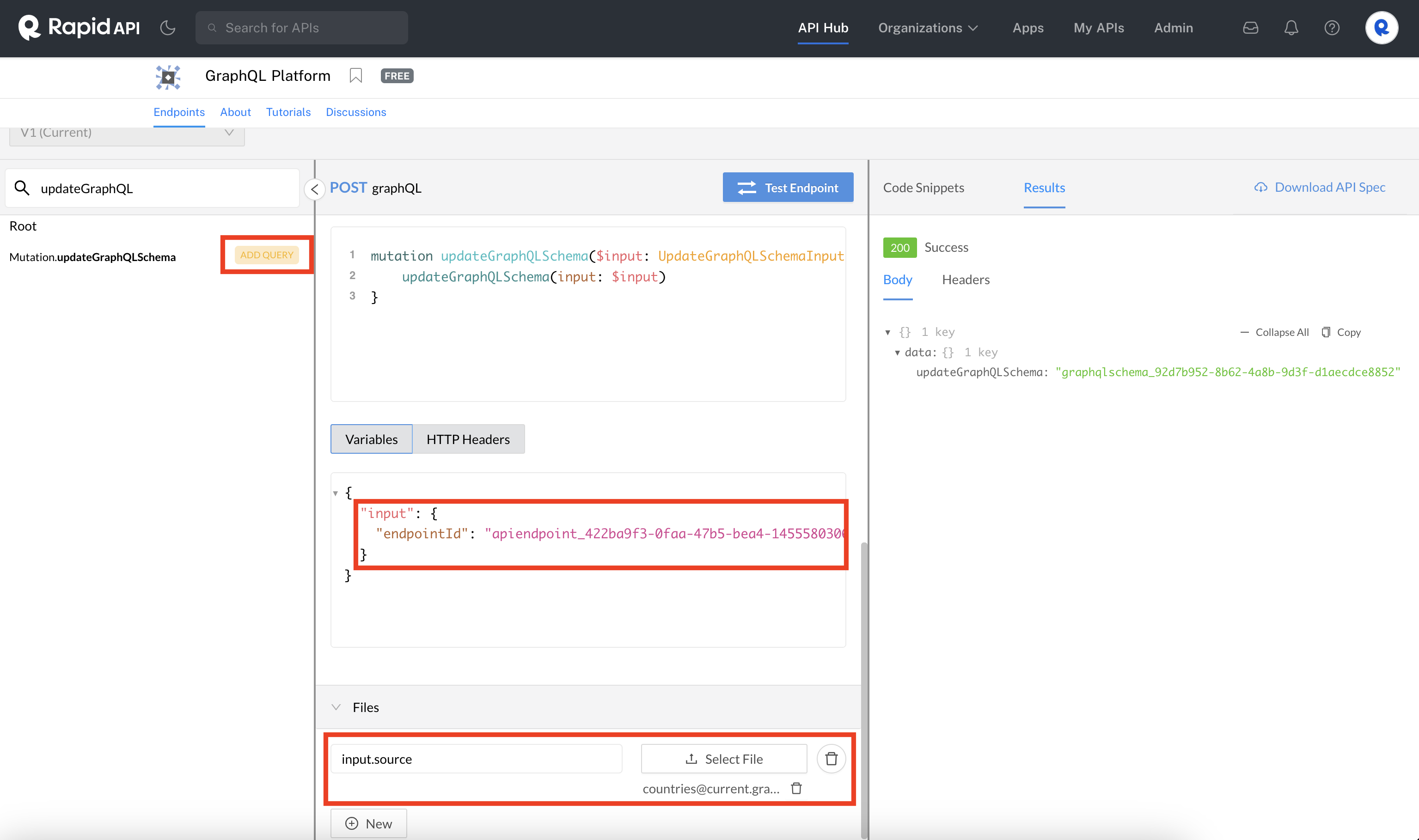
Executing the updateGraphQLSchema mutation in the API Hub playground.
The following sample Node.js Axios code executes the Mutation.updateGraphQLSchema
operation programmatically.
const FILENAME = "simpleschema-updated.graphql"; // replace with your file
const URL = "https://[YOUR URL].p.rapidapi.com/";
const HOST = "[YOUR HOST].rapidapi.com";
const TEAMORUSERKEY = "[YOUR ID]";
const USERKEY = "[YOUR ID]"; // needed if a team owns the API
const ENDPOINTID = "[YOUR ENDPOINT]";
const MUTATION = `
mutation updateGraphQLSchema($input: UpdateGraphQLSchemaInput!) {
updateGraphQLSchema(input: $input)
}
`;
const axios = require("axios");
const FormData = require("form-data");
const fs = require("fs");
const formData = new FormData();
formData.append(
"operations",
JSON.stringify({
query: MUTATION,
variables: {
input: { endpointId: ENDPOINTID },
},
})
);
formData.append("map", JSON.stringify({ 0: ["variables.input.source"] }));
let file = fs.readFileSync(FILENAME);
formData.append("0", file);
axios
.post(URL, formData, {
headers: {
...formData.getHeaders(),
"Content-Length": formData.getLengthSync(),
"x-rapidapi-host": HOST,
"x-rapidapi-key": TEAMORUSERKEY,
"x-rapidapi-identity-key": USERKEY,
},
})
.then(function (response) {
console.log(response.data);
})
.catch(function (error) {
console.error(error.response.data);
});
Create a major version of a GraphQL API using introspection
Use the createGqlApiVersions
mutation to create a major version of an existing GraphQL API using introspection to populate the GraphQL schema.
In Variables, specify the API ID of the API for which you want to create a major version. Specify the version's name
and introspectionCallUrl
. Set isIntrospectionCall
to true.
mutation CreateGqlApiVersions($gqlApiVersionCreateInput: [GqlApiVersionCreateInput!]!) {
createGqlApiVersions(gqlApiVersions: $gqlApiVersionCreateInput) {
id
versionStatus
}
}
{
"gqlApiVersionCreateInput": {
"api": "YOUR API ID",
"name": "v3",
"introspectionCallUrl": "YOUR BASE URL",
"isIntrospectionCall": true
}
}
Updating the schema for a major version of a GraphQL API
Since each version's endpoint ids are unique, you can update the GraphQL schema for a major API version by following the instructions to Update a GraphQL API's schema, specifying the endpoint id for the major version.
Updated 5 days ago